Predictive Code Completion in Xcode
Getting started & first impressions
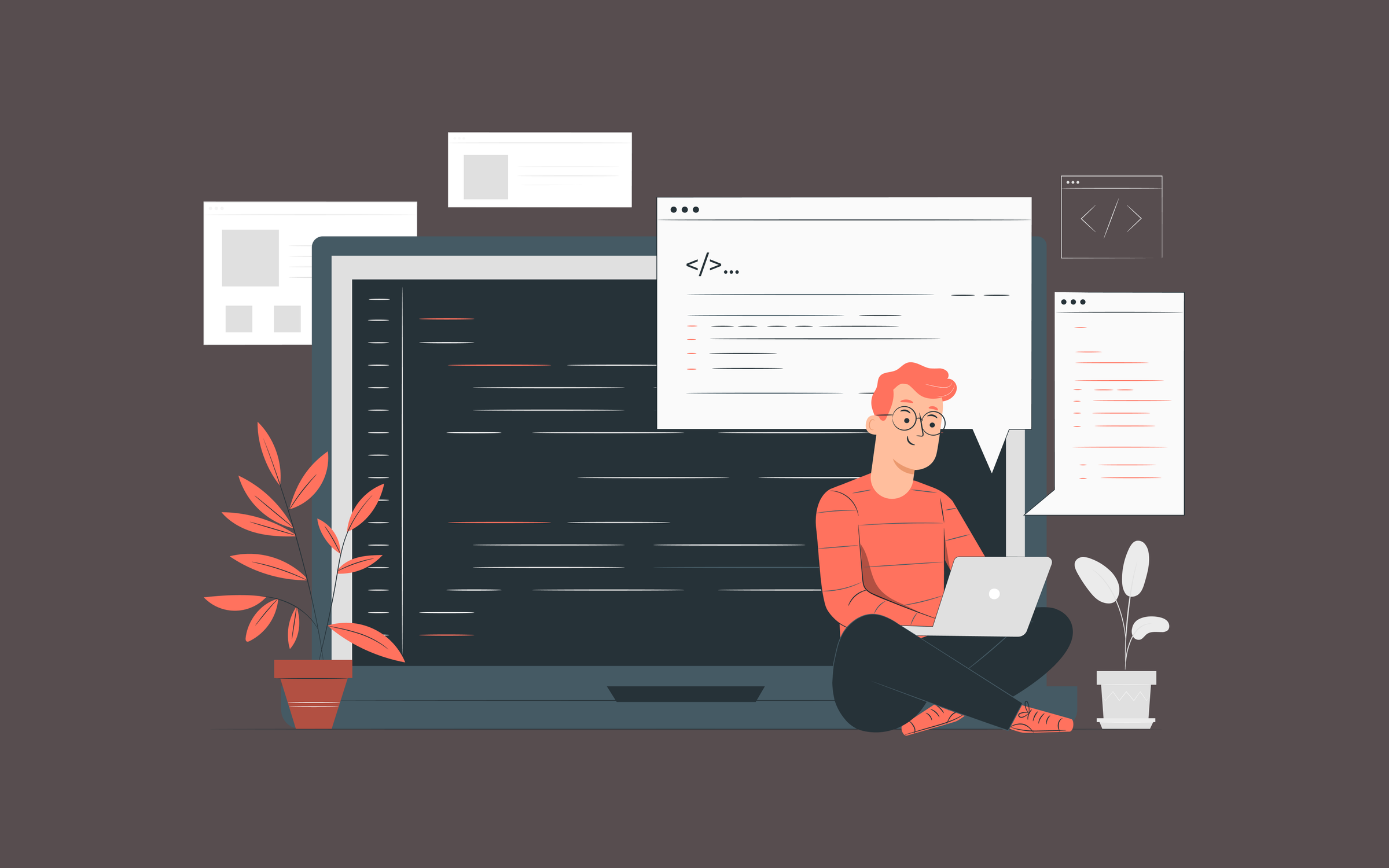
Like it or not, AI assistance in software development is here to stay for the foreseeable future. If you’ve embraced technologies like ChatGPT to answer coding questions, format mock data, or take the first crack at writing a new feature, you’ve likely seen an overall boost to your productivity. If you’re a Swift developer and you haven’t dipped your toes in just yet — either due to privacy and security concerns, or mixed results with generative AI in other contexts — you may want to take another look, as major players have now entered the ring that offer AI assistance directly within Xcode.
This year, Apple’s first-party offering, its privacy-focused Xcode Code Completion model was introduced, and is now out of beta. Following this, GitHub announced a public preview of Copilot for Xcode, ChatGPT opened an early beta of its Works with Apps feature that is Xcode-compatible, and Cursor (albeit in a separate IDE) is gaining steam in the community. In this post, we’ll look specifically at Xcode’s new code completion model, how to set it up, and what it can do to improve your productivity.
Prerequisites
To take advantage of Xcode’s predictive code completion, you’ll need to be up-to-date software-wise. The feature was introduced first in Xcode 16, and while Xcode 16 runs just fine on macOS Sonoma, the latest version of macOS (Sequoia) is required to use the code completion feature. Hardware-wise, you’ll need an Apple Silicon-based computer and at least 8 GB of memory, in addition to about 2 GB of additional disk space for the model itself.
So in summary, that’s:
- macOS Sequoia or later
- Xcode 16 or later
- Apple Silicon-based computer
- 8 GB of memory
- 2 GB of free disk space
Installation
Xcode’s predictive code completion feature has all the advantages of being a first-party solution, including being dead simple to install. When launching Xcode 16 or later for the first time, you’ll see a window that allows you to select components to install right away. Check Predictive Code Completion Model and any platforms you’ll be using before clicking Download & Install.
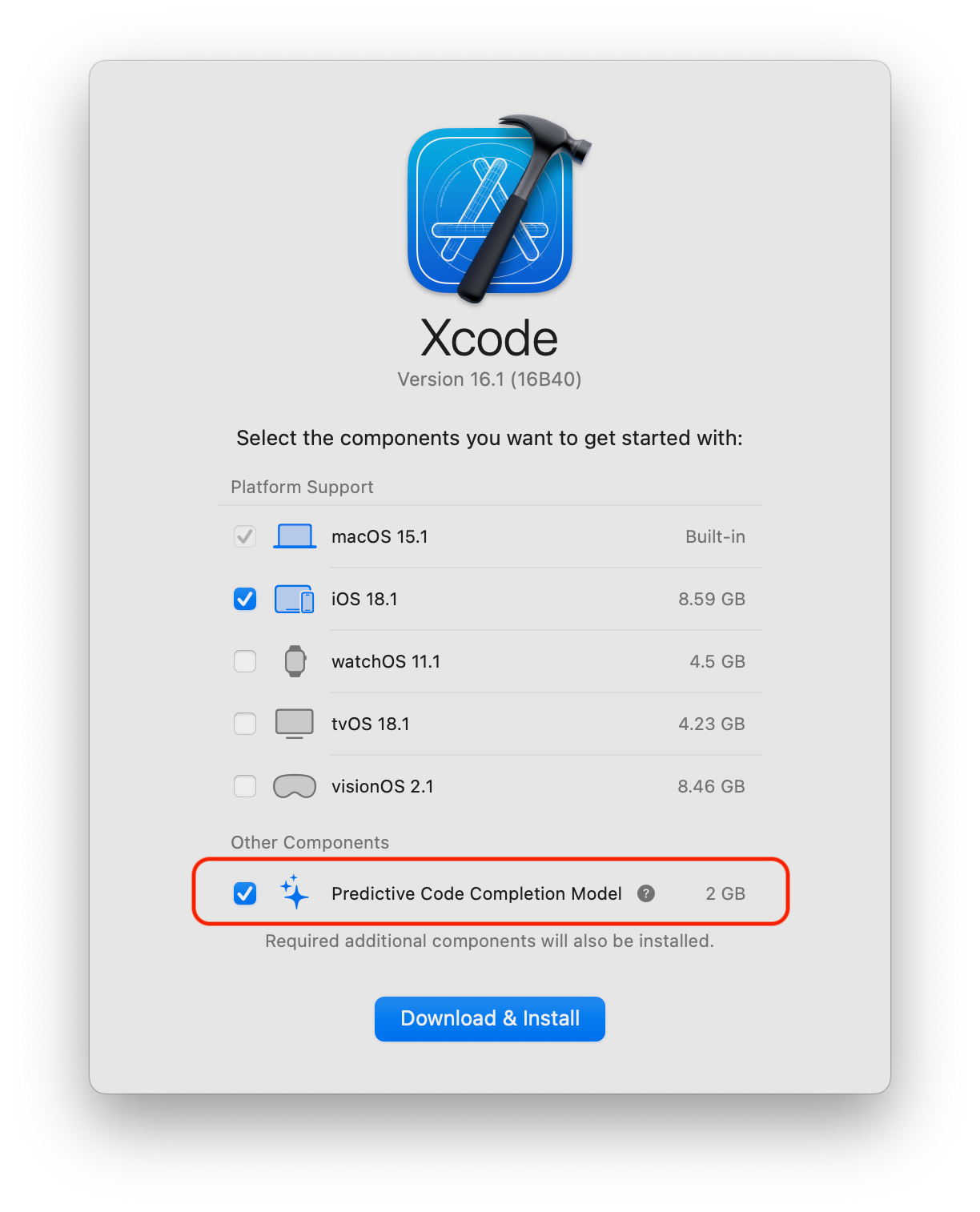
Xcode’s first launch window, which includes a checkbox for downloading the predictive code completion model.
Don’t worry if you’ve opted not to download this on first launch. Simply navigate to Settings → Components → Predictive Code Completion Model, and click the Get button to download and install the model.
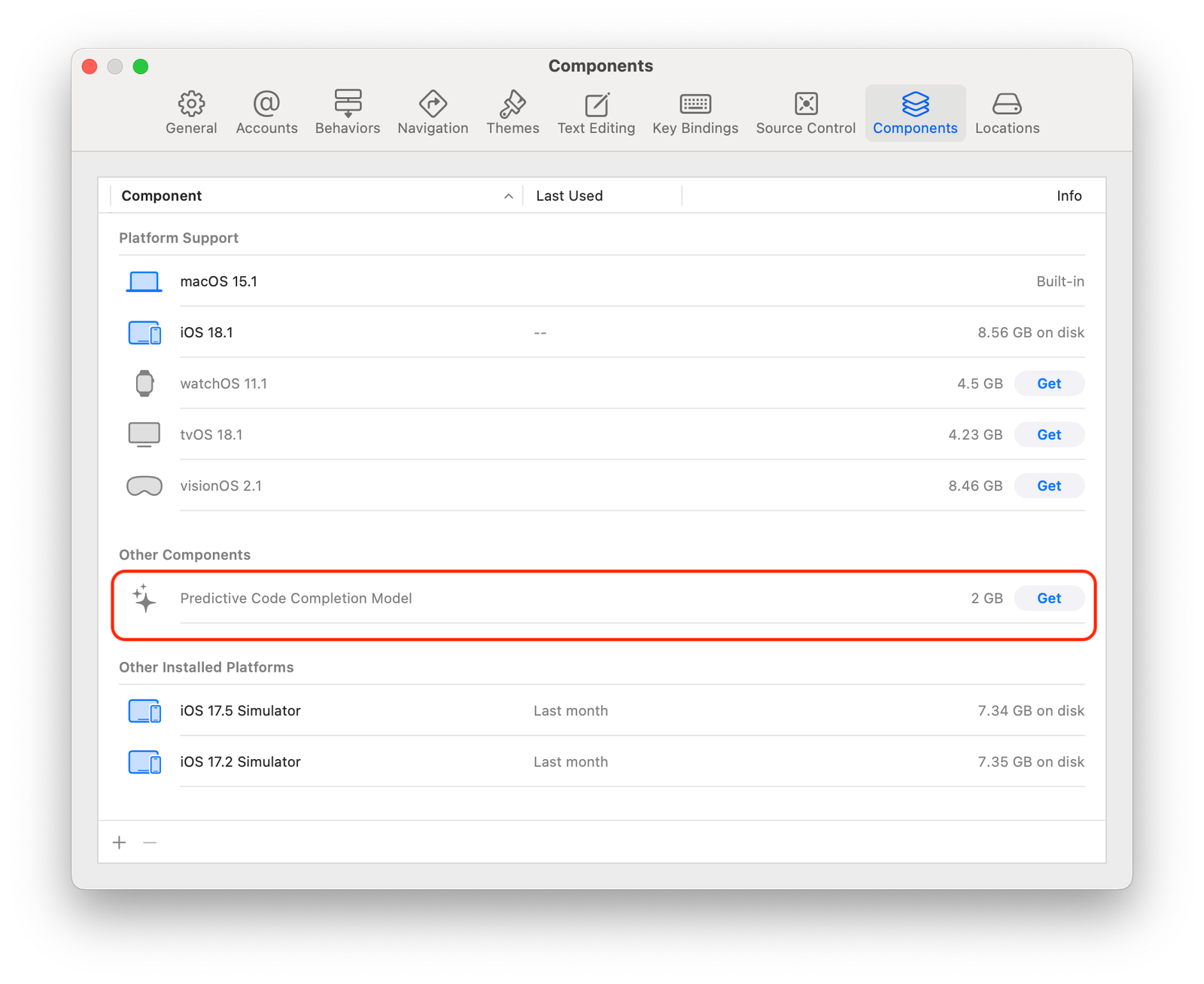
And that’s it! You can turn the feature off at any time by navigating to Settings → Text Editing → Editing and unchecking Predictive code completion.
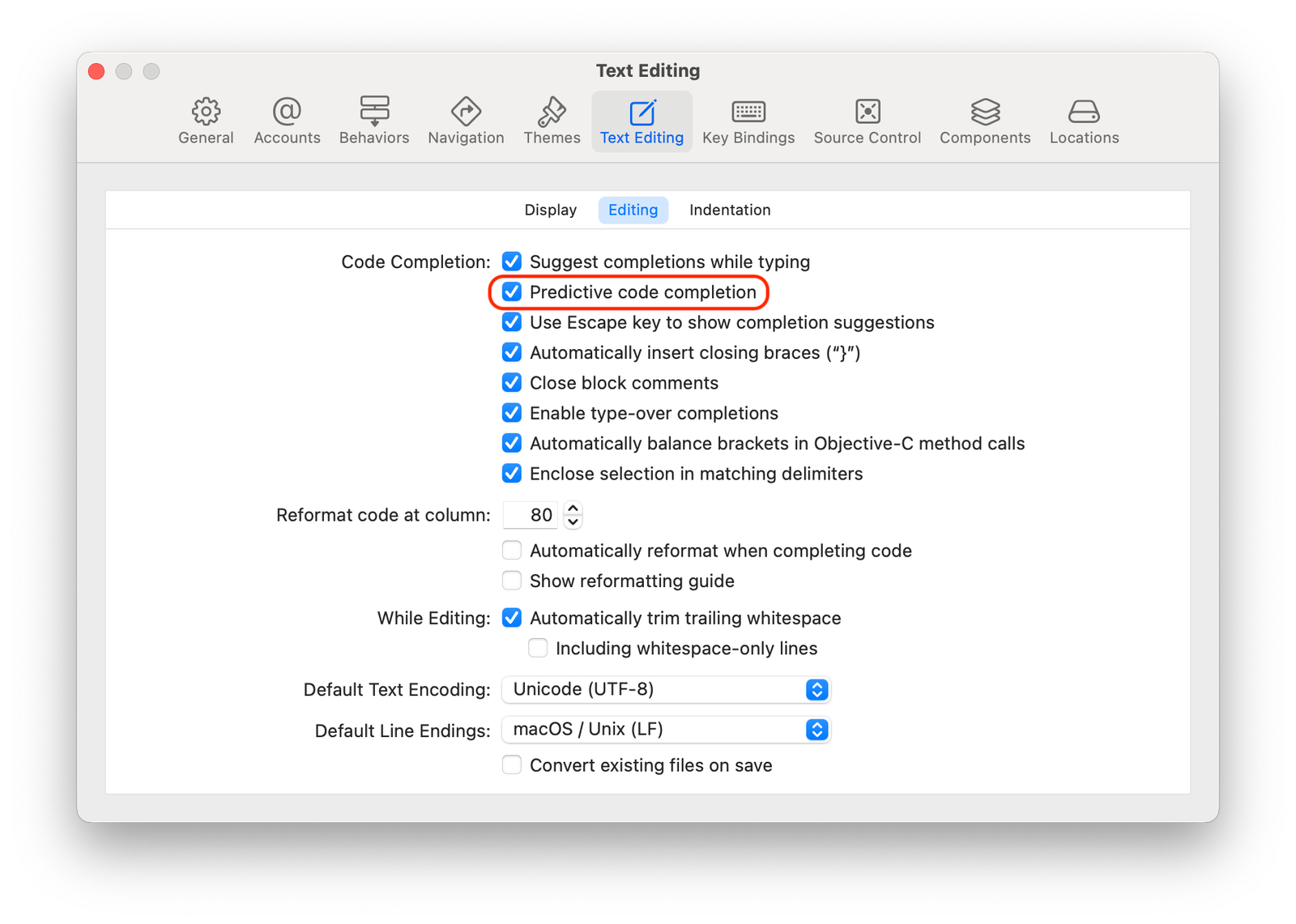
Code completion in action
To take this new feature for a spin, we wrote an implementation of a “take home” code exercise that we’ve given engineering team candidates in the past. Nothing too complex:
- Hit a specific endpoint to download photos
- Display those photos in a scrolling grid
Immediately, we can see that Xcode’s predictive code completion has clear advantages to being built in, compared to the other AI-assisted code features we’ve tried. There will always be a barrier for third-parties to achieve what Apple can do, since they’re not bound by the limitations of Xcode extensions, or the need to run in an entirely separate interface. Xcode’s suggestions appear while typing, similar to snippets, completing the current line and suggesting more of an implementation to follow (truncated with “…”):
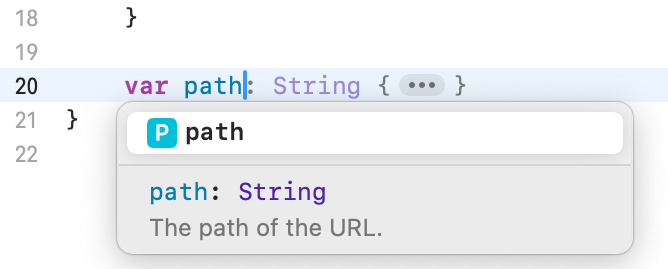
Xcode’s predictive code completion suggesting the type of a computed path
variable declaration from a protocol. The implementation of the property is truncated with “…”
One stroke of the tab
key will complete the line, and may suggest the body or implementation of the declaration:
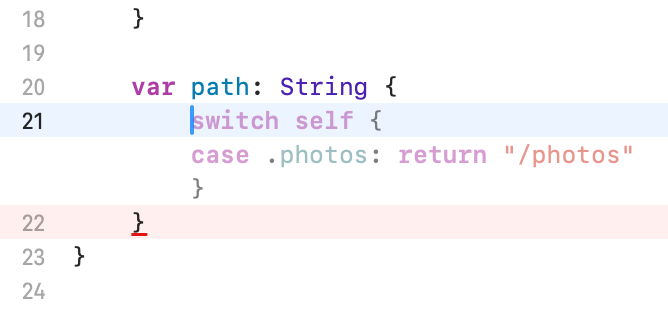
Xcode’s predictive code completion suggesting the body of the computed path property.
If you’re satisfied with the suggestion, or if it provides a good enough starting point, one more tab
will accept it.
Code predictions blend fairly well into your coding interface. However, I’ve found that they can be a bit visually distracting when you stop to think about what you’re typing and aren’t looking for suggestions. Constantly turning the feature on and off when you need vs. don’t need it is a non-starter for me, so I like to leave it on, and hope I’ll get used to the additional visual noise.
Suggestion quality
No AI-assisted coding tools can read your mind, and I highly recommend using them only as a start to get something working, and then refine the code manually. They’ll produce erroneous code, off-the-wall suggestions, and use bad practices at times. Xcode’s new code completion model is no exception — we shouldn’t expect it to get things perfect every time. It’s amazing that it works at all, but still, it can get frustrating to use if you expect too much.
Overall, I haven’t found it useful to rely on predictive suggestions for top-level declarations. Even with strict patterns in place in a codebase, and even with types that declare conformance to existing protocols, I very rarely find the suggestions useful. Again, it can’t read your mind, but you can’t be blamed for expecting more when there’s a lot of context to be gleaned from the rest of your codebase. For example, our Store
protocol has several required properties and methods, and each store implementation follows a convention of a single @Published
state
property. That’s just too much to ask at this time, and we’d be better off simply using Xcode’s “fix-it” suggestions to add protocol stubs.
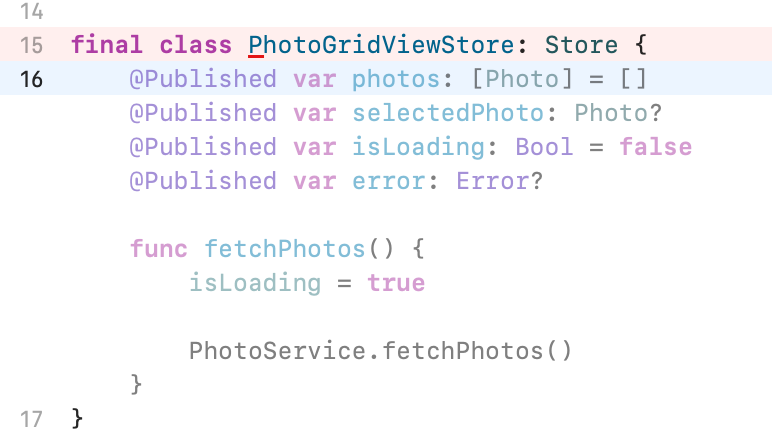
Xcode’s predictive code completion suggesting a workable start to a “store” class, but failing to follow patterns already set forth in the codebase.
Additionally, without more context in a Swift file than the default header, we frequently see Xcode’s predictive code completion try to introduce tests where they don’t belong. In this example, we’re trying to create a Photo
type in a project with no prior test module whatsoever:
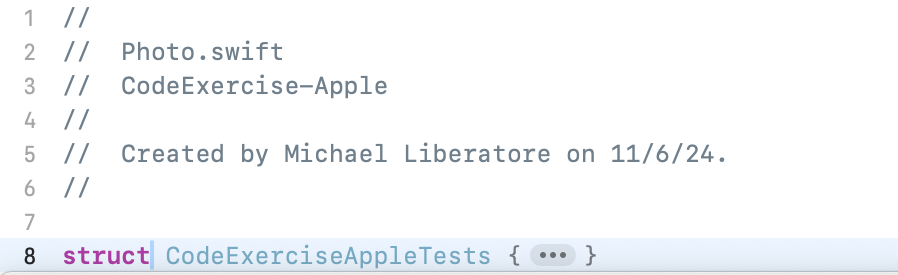
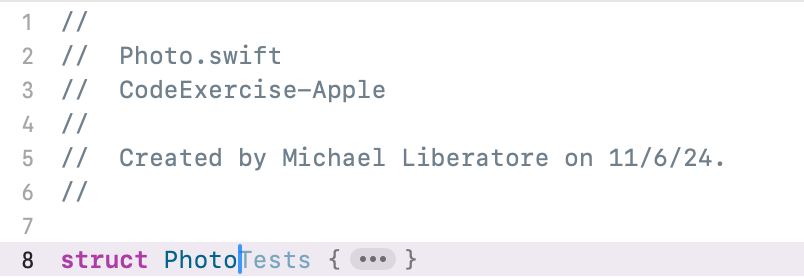
Xcode’s predictive code completion hellbent on suggesting test declarations in a file named Photo.swift
.
We have a lot more success applying suggestions to small, missing implementations, and working carefully to refine them.
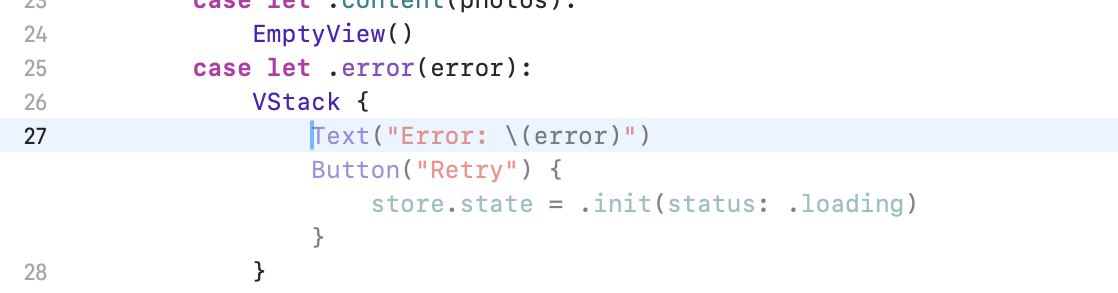
Xcode’s predictive code completion suggesting a Text
displaying an Error
and a Button
to retry a network request within a SwiftUI view. It’s a good starting point.
Frequently, even within some quality suggestions, Xcode suggests snippets that include symbols that don’t exist. I don’t see this as too much of a problem, as in simple use cases, the suggestions tend to include types that simply don’t exist yet, and can somewhat serve as a suggestion to create them. Still, it’d be nice if it were sophisticated enough to create them for you. In the example below, the type PhotoRow
doesn’t exist yet, but our properly factored final implementation of a photo list would likely use a specific type to wrap the layout of individual photos.
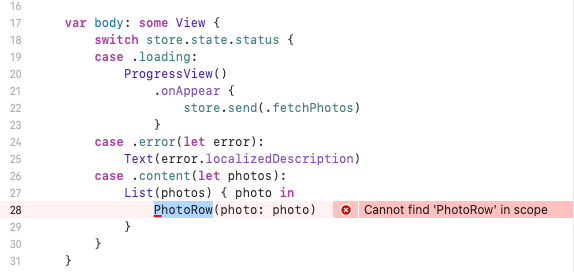
An accepted suggestion of a scrolling list of PhotoRow
s, a type that doesn’t exist in our codebase.
Xcode’s suggestions can be improved by pre-commenting expected logic in plain English. By adding a comment specifically mentioning “grid” and the number of columns, we get closer to the actual structure we want.

An early refinement attempt at getting Xcode’s predictive code completion to create a scrolling grid of photos. Xcode suggests a LazyHGrid
initialized with columns
. That API does not exist. Additionally, a parameter on the same line is not given a value.
With enough refinement of the comments, we can usually get Xcode to suggest something workable, but in simple cases like this that require multiple attempts, it’s hard to say whether we’re saving any time vs. writing the code ourselves.
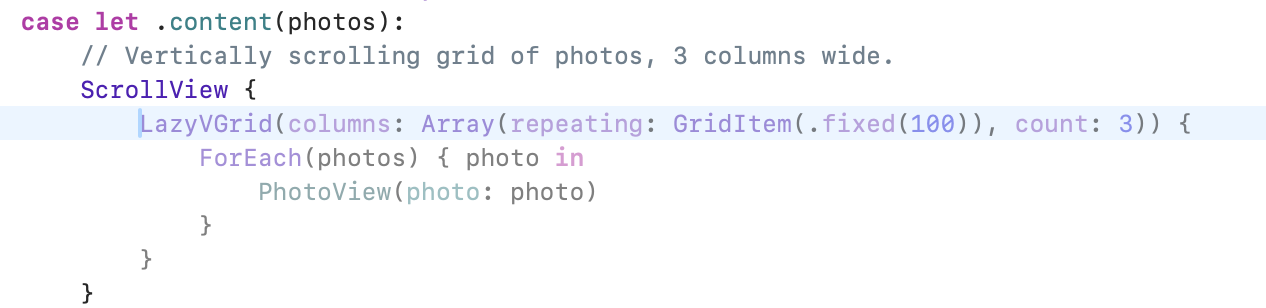
By specifying “vertically scrolling grid” and “3 columns wide,” in our comment we were able to get Xcode to suggest a quality start to our photo grid.
The future
At the time of writing, Xcode’s built-in code completion model is far more limited in functionality than AI-assisted tools that work with other IDEs. But even if we’re late to the party as Apple platform developers, the future is looking bright. At WWDC24, Apple announced that Swift Assist would be coming later this year — Swift Assist provides an in-editor text field for the developer to make arbitrary requests to write code for you, with an emphasis on prototyping.
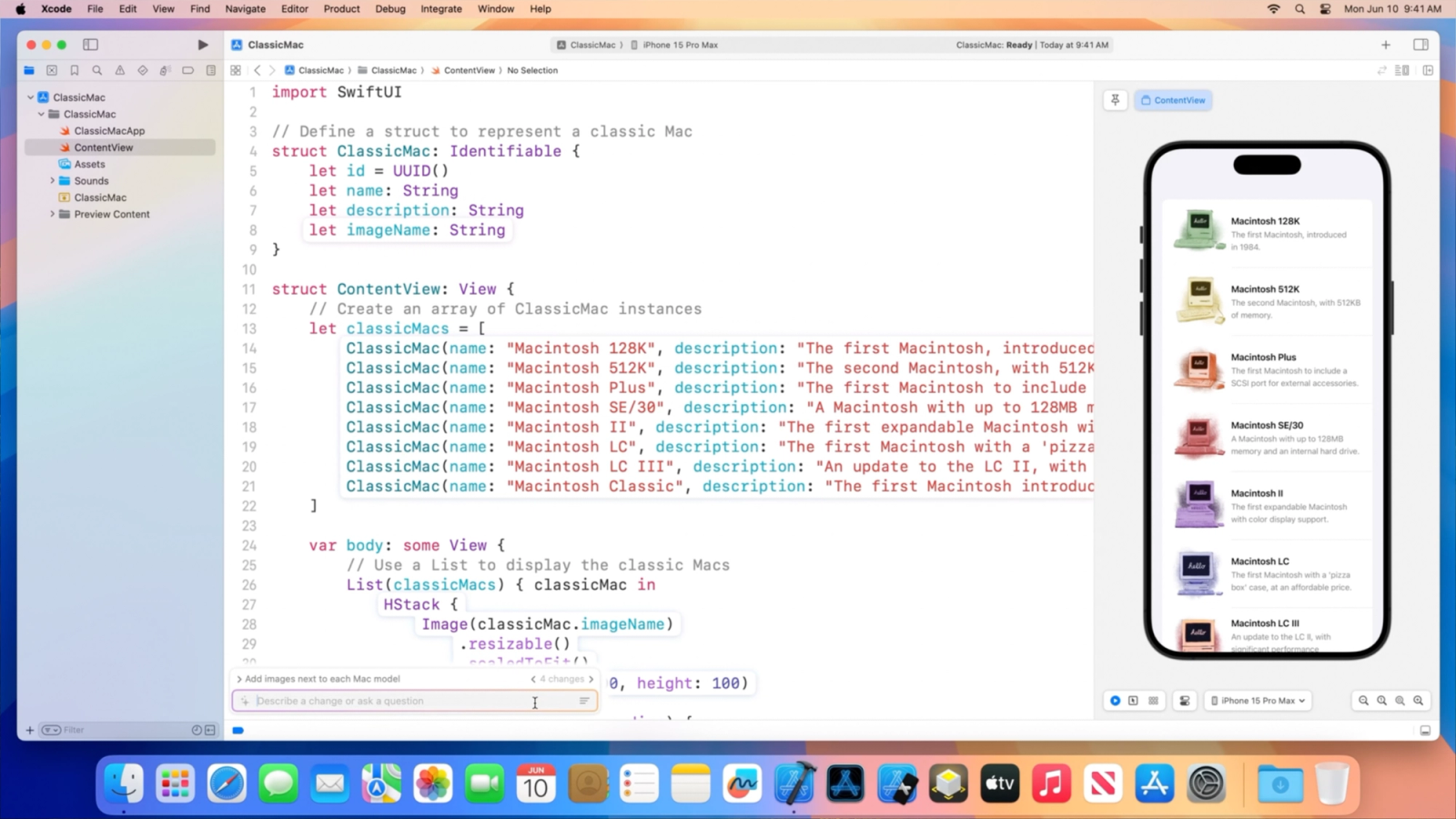
Screenshot demoing Swift Assist from Apple’s WWDC24 during Platforms State of the Union.
Is it worth using now?
I think so! For the time being, I’ll be using a mix of Xcode’s code completion for the basics, and I’ll keep ChatGPT in my dock and at-the-ready for broader and more complex assistance. Over time, I hope to be able to lean first-party, and use only Xcode’s built-in features. With Apple’s outsized focus on privacy and security, and the built-in nature of the features, I could look past some small shortcomings for the benefits of convenience. The pace at which competitors in the space are improving for other languages and IDEs does make me worry that Apple will struggle to keep up, but that remains to be seen.